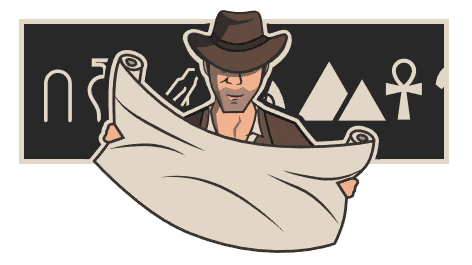
In this example we will show how to implement the component scrolling via mouse drag & drop like Google Map, Google Earth, Figma etc.
To implement the scrolling we will use the react-indiana-drag-scroll
library which is great library to Implement scroll on drag.
So let’s get started.
Demo
Try it yourself! Go to demo website / sandbox
Install
npm install --save react-indiana-drag-scroll
or install via yarn
yarn add react-indiana-drag-scroll
Usage
import React, { Component } from 'react';
import ScrollContainer from 'react-indiana-drag-scroll';
class Example extends Component {
render () {
return (
<ScrollContainer className="scroll-container">
{ ... }
</ScrollContainer>
)
}
}
Component properties
Prop | Type | Description | Default |
---|---|---|---|
vertical | Bool | Allow vertical drag scrolling | true |
horizontal | Bool | Allow horizontal drag scrolling | true |
hideScrollbars | Bool | Hide the scrollbars | true |
activationDistance | Number | The distance that distinguish click and drag start | 10 |
children | Node | The content of scrolling container | |
onScroll | Function | Invoked when user scrolling container | |
onEndScroll | Function | Invoked when user ends scrolling container | |
onStartScroll | Function | Invoked when user starts scrolling container | |
onClick | Function | Invoked when user clicks the scrolling container without dragging | |
component | String | The component used for the root node. | ‘div’ |
className | String | The custom classname for the container | |
style | Number | The custom styles for the container | |
innerRef | ElementType | The ref to the root node (experimental alternative to getElement ) | |
ignoreElements | String | Selector for elements that should not trigger the scrolling behaviour (for example, ".modal, dialog" or "*[prevent-drag-scroll]" ) | |
nativeMobileScroll | Bool | Use native mobile drag scroll for mobile devices | true |
Static functions
Name | Returns | Description |
---|---|---|
getElement | HTMLElement | Returns the HTML element |
How to set the initial scroll?
To set initial scroll you need get the ref to the root node of the ScrollContainer
. It can be implemented by using innerRef
property or the static function getElement
. At the worst you can use the ReactDOM.findDOMNode
method.
License
The source code is licensed under MIT, all images (except hieroglyphs) are copyrighted to their respective owner © Norserium
That’s it. Feel free to leave a comment below.